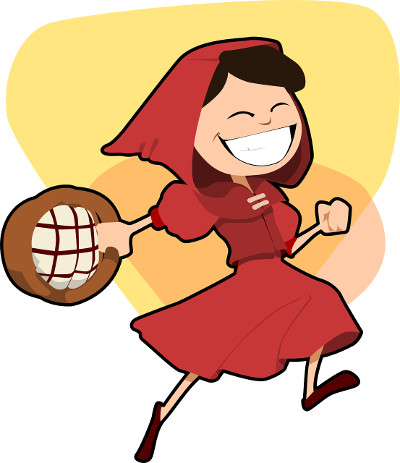
Once upon a time, there was a little girl who always wore a red riding cloak. So everyone called her Little Red Riding Hood. One moning the mother said to the child: "Red Riding Hood, today is your grandmother's birthday. Bake her favorite cake, take a bottle of good old wine from the cellar, put everything in one basket, and go visit her."
Help Little Red Riding Hood bake a cake! Doing this you will expand your knowledge about functions.
Functions with Parameters
With parameters, values can be passed to a function. In the following function you can specify how many steps the player should take:
multiMove(int steps) { int i = 0; while (i < steps) { move(); i = i + 1; } }
To go 5 steps just put the number 5 between the parentheses:
multiMove(5);
To invoke a function with multiple parameters, the values are separated by commas.
drawRectangle(21, 4);
Explanations
- In previous programs it was rather cumbersome to always write the parentheses
()
for each function. Now it is clear that the previously used functions were merely cases where no parameter was passed. - Inside the parentheses of a function declaration, the parameter (in this case
steps
) is specified with a type (hereint
). Multiple parameters are separated by commas as seen in this example:
drawRectangle(int width, int height)
- When such a function is called, the values are copied into the variables (in this case into
width
andheight
).
Baking a Cake
We will now use our player to “bake” a birthday cake for the grandmother. The cake will be made of stars.
TASK 5.01: Baking a Cake
In preparation for baking, the following methods should be provided:
-
turnAround()
Rotates the player by 180 degrees. -
multiMove(int steps)
The player takes the number of steps in the current direction (see example in the theory section above). -
putStars(int count)
The player puts the specified number of stars. The first one he places at the current position, the other following in his line of sight.
The player is to draw a rectangle with stars symbolizing the cake.
The player starts in the lower left corner and looks to the right. The player should be able to make a rectangle with variable width and height! The function call drawRectangle(21, 4)
should therefore create a rectangle with a width of 31 and height of 4.
TASK 5.02: Candles on Cake
To make the cake look like a birthday cake it needs a few candles, of course. Extend your program with an additional method drawCandles(int count)
which sets the specified number of candles on the cake.
Function with Return Values
A function can return a value as a result. The following function will multiply the specified number
by two and return the result:
int twoTimes(int number) { return 2 * number; }
The result can either be stored in a variable or directly be used as follows:
multiMove(twoTimes(4));
Explanations
- The type of the return value (in this case
int
) is specified before the name of the function. If the function does not return a value, we writevoid
. - To return a value we write
return
followed by the value.
TASK 5.03: Candles for Age
We want to put on the cake a candle for every decade of grandmother’s age. As Little Red Riding Hood does not know exactly how old her grandmother is, she must first ask her mother.
Program a function called howOldIsGrandma()
that returns the age. With the help of this function the rest of the program should place a candle on the cake for every decade.
double
. To get an int
again, we can use the toInt()
or round()
functions. Example:
(5 / 3).toInt()
TASK 5.04 (difficult): Layered Cake
Let the player “bake” a layered cake for the grandmother. The player should add a layer for every decade that the grandmother is over 50. Each layer should have two lines and should be indented on both sides by two stars.
What’s next?
Learn about the next steps.
Credits
Planet Cute images by Daniel Cook (Lostgarden.com), published under CC BY 3.0.
Oleg Yadrov improved the “Planet Cute” images and was so kind to let me use them. The images were optimized with the great TexturePacker.
Some exercises in Hello Dart
were inspired by Kara. Kara was developed by Jürg Nievergelt, Werner Hartmann, Raimond Reichert et. al.